Welcome, Guest |
You have to register before you can post on our site.
|
Latest Threads |
QID:EC1223
Forum: CCNP ENCOR 350-401 Forum
Last Post: help_desk
Yesterday, 11:07 AM
» Replies: 1
» Views: 95
|
QID:EC708
Forum: CCNP ENCOR 350-401 Forum
Last Post: chewosaurus
06-13-2025, 01:41 PM
» Replies: 3
» Views: 664
|
QID:EC082
Forum: CCNP ENCOR 350-401 Forum
Last Post: help_desk
06-11-2025, 10:14 AM
» Replies: 1
» Views: 256
|
QID:EC172
Forum: CCNP ENCOR 350-401 Forum
Last Post: help_desk
06-11-2025, 09:48 AM
» Replies: 1
» Views: 256
|
I PASSED!
Forum: CCNP ENARSI 300-410 Forum
Last Post: help_desk
06-03-2025, 12:26 PM
» Replies: 1
» Views: 601
|
AR517 AR683 Duplicate que...
Forum: CCNP ENARSI 300-410 Forum
Last Post: bonz86
05-31-2025, 07:37 AM
» Replies: 0
» Views: 427
|
AR507 and AR667 same ques...
Forum: CCNP ENARSI 300-410 Forum
Last Post: bonz86
05-31-2025, 04:46 AM
» Replies: 0
» Views: 415
|
QID:EC021
Forum: CCNP ENCOR 350-401 Forum
Last Post: help_desk
05-28-2025, 01:26 PM
» Replies: 1
» Views: 662
|
QID:EC956
Forum: CCNP ENCOR 350-401 Forum
Last Post: help_desk
05-26-2025, 02:19 PM
» Replies: 2
» Views: 1,023
|
Failed again
Forum: CCNP ENCOR 350-401 Forum
Last Post: chewosaurus
05-24-2025, 05:03 PM
» Replies: 2
» Views: 973
|
|
|
QID:EC082 |
Posted by: chewosaurus - 06-09-2025, 02:16 PM - Forum: CCNP ENCOR 350-401 Forum
- Replies (1)
|
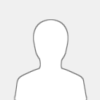 |
I have an issue with "propriety syntax in configuration files based on Ruby" being allocated to Chef.
Why:
Because Chef uses plain ruby whereas puppet uses DSL. The wording "based on ruby" implies that chef isn't using "pure/plain" ruby instead it's using a branch of ruby which more accurately describes what puppet uses, that being DSL inspired by Ruby but not actually ruby.
Thanks
|
|
|
QID:EC172 |
Posted by: chewosaurus - 06-09-2025, 11:52 AM - Forum: CCNP ENCOR 350-401 Forum
- Replies (1)
|
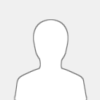 |
The correct answer is currently "A NETCONF request was made for a data model that does not exist".
Why I feel this is not accurate:
An empty <data/> element tells the administrator: “Your query succeeded, but there’s nothing under the subtree you asked for.”
It does not indicate that the data model itself is missing.
RFC 6241 §7.1, second paragraph: “If the server has no data that matches the selection criteria… it MUST reply with an empty <data/> element.”
Maybe the answer should be along the lines of "A NETCONF request was made for data that does not exist".
Thanks
|
|
|
QID:EC708 |
Posted by: chewosaurus - 06-04-2025, 04:44 PM - Forum: CCNP ENCOR 350-401 Forum
- Replies (3)
|
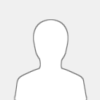 |
I believe the answer "If a router with a higher IP address and the same priority as the active router becomes available, that router becomes the new active router 5 seconds later.” is incorrect for this reasoning:
Even in the case of pre-emption on the new router -
Pre-emption triggers only when the newcomer’s priority is higher than the current active router. A higher IP address breaks a tie during the initial election, but it does not pre-empt an already-active router whose priority is equal. The 5-second delay therefore would not apply in that equal-priority scenario.
|
|
|
I PASSED! |
Posted by: bonz86 - 06-02-2025, 07:32 PM - Forum: CCNP ENARSI 300-410 Forum
- Replies (1)
|
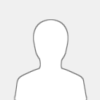 |
First attempt and passed! 6/2/2025
I got 48 MCQ, 1 drag and drop, and 3 sims.
Sims are spot on, I got the Ipsec v2, the telnet, and timestamps sims.
There were about 10 new questions that were not in H2P but if you study the material on top of H2P you won't have issues passing. Study and do the sims and the test will be a breeze.
I prepped for about 2 months before my exam and I went through all the h2p questions and sims and did not stop until I was able to almost recite every question and answer. For topics I did not know I went and did the research and studied the concepts.
I have always used h2p ever since college and I have passed all exams first try from CCNA to the old CCNP (3 test) to the new Encore and now Enarsi exams.
Goodluck to all future test takers!
|
|
|
AR507 and AR667 same question |
Posted by: bonz86 - 05-31-2025, 04:46 AM - Forum: CCNP ENARSI 300-410 Forum
- No Replies
|
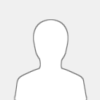 |
AR507 and AR667 are the same question but give two different answers.
I would think that LDP is the correct answer given the explanation. AR507 states MP-BGP is the answer but is not correct.
|
|
|
PASSED! |
Posted by: mountfestus - 05-22-2025, 04:47 PM - Forum: CCNP ENCOR 350-401 Forum
- Replies (3)
|
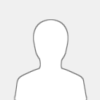 |
I passed my ENCOR 350-401 exam today. There were quite a number of new questions. At one point during the exam, I thought I would fail; however, the six simulation labs were all spot on.
|
|
|
|